This tutorial walks you through some very common arcpy.mapping workflows and introduces some important concepts along the way. It is aimed to help users that are completely new to Python scripting and arcpy.mapping. The steps are generic enough to work with any data and all license levels.
Note:
The only requirement is that you have a feature layer file (.lyr) before starting the tutorial.
The steps below present a very high-level overview of arcpy.mapping. Throughout the tutorial, there are links to detailed help topics that provide much more information about the concepts involved, and in nearly all cases, the topics include additional sample snippets of code.
Python window
The easiest place to start learning arcpy.mapping is in the Python window. The Python window is part of the ArcMap framework and provides autocompletion so you can easily read the names and order of the function parameters. The steps below show you how to set up the Python window for a satisfactory end-user experience and also to match the screen captures throughout this document.
- Open a new, empty map document in ArcMap.
- Click Geoprocessing > Python.
- Click the title bar of the Python window and hold down your left mouse button.
- Drag the Python window to the docking anchor located at the bottom of the ArcMap application and release the button.
- Click in the code pane (with the >>> characters) of the Python window. You should see some help text appear in the help pane to the right.
- Right-click the code pane and choose Help Placement > Bottom. Now the help should appear below the code pane. You may need to adjust the divider between the two panes so there is enough space to type code and see the help.
- Now type the following statement (be careful, Python is case sensitive):
- Right-click the code pane and click Clear All to clear the code pane.
If it is your first time opening the Python window, it appears as floating over the application. The next steps are to dock the Python window and modify the help placement.
>>> arcpy.mapping.ExportToPDF(
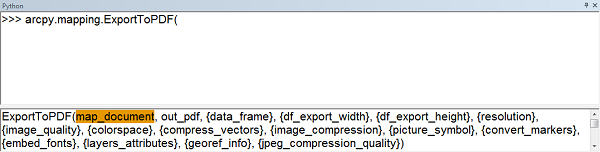
Referencing an existing map document
Typically, one of the first operations you do with an arcpy.mapping script is reference an existing map document (.mxd) or layer file (.lyr) that you want to do something with. In this section, you'll reference a map document.
There are two ways to reference a map document. The first is to reference it on disk by providing a path to the .mxd file. If you are building a script that is to be run outside the ArcGIS environment, you must reference a map document using its path. The second way is to reference the map document that is currently loaded into the ArcMap application (in this case, your untitled.mxd). When working in the Python window within ArcMap, it is convenient to reference the currently loaded map document because changes made to it can be seen directly in the application. The following steps will reference the map document currently loaded into ArcMap.
- In the Python window, type the following and press ENTER when done:
- In the Python window, type the following:
- In the Python window, type the following and press ENTER when done:
- In ArcMap, click File > Map Document Properties.
- Click Cancel when done with the Map Document Properties dialog box.
- In the Python window, type the following and press ENTER when done:
- In the Python window, type the following and press ENTER when done:
>>> mxd = arcpy.mapping.MapDocument("CURRENT")
>>> mxd.
>>> mxd.author = "YOUR NAME GOES HERE"
>>> mxd.save()
>>> print mxd.filePath
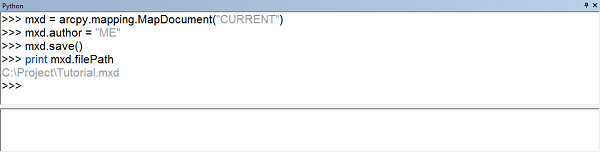
Add a layer file to the map document
Now that you have a reference to a map document, the next thing you will do is add a layer file (.lyr) to the map document. This can be done with the arcpy.mapping AddLayerfunction.
- In the Python window, type the following:
- In the Python window, backspace to remove the AddLayer function, type the following, then press ENTER when done:
- In the Python window, type the following and press ENTER when done. The path you provide will most likely be different than the example below.
- In the Python window, type the following and press ENTER when done:
>>> arcpy.mapping.AddLayer(
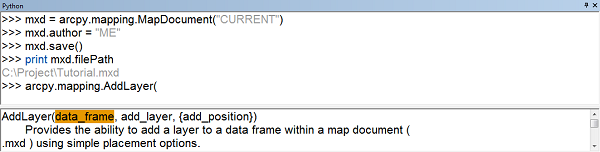
>>> df = arcpy.mapping.ListDataFrames(mxd, "Layers")[0]
Note:
For the following steps, you need to locate an existing layer file. If you don't have one, you will need to author one.
>>> lyrFile = arcpy.mapping.Layer(r"C:\Project\data\Rivers.lyr")
>>> arcpy.mapping.AddLayer(df, lyrFile)
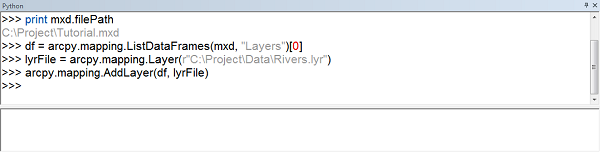
Export a map document to PDF
Exporting a map document to PDF is incredibly easy and only requires a single line of code. You already saw the syntax for the ExportToPDF function near the beginning of the tutorial. Now you will complete the syntax.
- In the Python window, type the following and press ENTER when done. The path you provide will most likely be different than the example below.
>>> arcpy.mapping.ExportToPDF(mxd, r"C:\Project\Doc1.pdf")
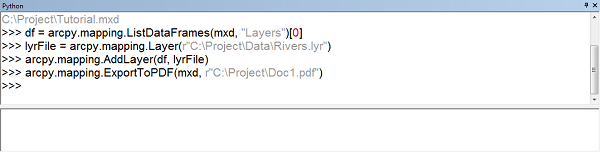
Reference a layer using the ListLayers function, change layer properties
There are many properties and methods available for working with layers in a map document. Earlier you added a layer to a map document from a layer file. The variable you created called lyrFilereferences the layer added to the map document. With this variable, you can modify some of the properties of the layer you added.
- In the Python window, type the following:
- In the Python window, backspace over the current text, type the following, then press ENTER:
- In the Python window, type the following two lines, pressing ENTER after each one:
- In the Python window, type the following two lines, pressing ENTER after each one:
>>> lyrFile.
>>> lyr = arcpy.mapping.ListLayers(mxd)[0]
>>> lyr.name = "Some New Name"
>>> lyr.visible = False
>>> arcpy.RefreshTOC()
>>> arcpy.RefreshActiveView()
Note:
If you are running stand-alone scripts outside ArcMap, these additional functions are not needed.
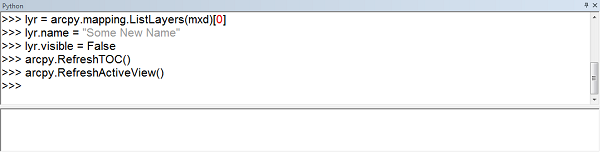
Change data frame extent
In this section you will change the data frame extent to match the extent of selected features. In a script, this would typically be done using a function like SelectlayerByAttribute. To simplify the experience, you will graphically select some features. First you'll need to turn your layer visibility back on using Python.
- In the Python window, type the following three lines, pressing ENTER after each one:
- In ArcMap, graphically select one or more features in your layer.
- In the Python window, type the following two lines, pressing ENTER after each one:
>>> lyr.visible = True
>>> arcpy.RefreshTOC()
>>> arcpy.RefreshActiveView()
>>> lyrExtent = lyr.getSelectedExtent()
>>> df.extent = lyrExtent
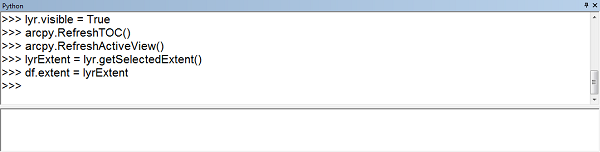
Export a map document to PDF (again)
You are going to export to PDF again. This time you are creating a second PDF document that will eventually be appended to a new document along with the first document.
- In the Python window, type the following and press ENTER when done. The path you provide will most likely be different than the example below.
>>> arcpy.mapping.ExportToPDF(mxd, r"C:\Project\Doc2.pdf")
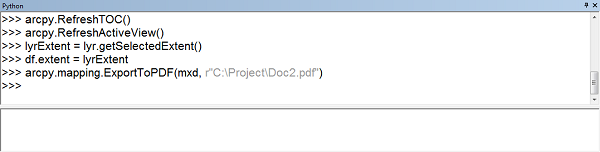
Create a new PDF document and append your two pages
The arcpy.mapping module contains some PDF document management functions that are perfect for creating multiple-page documents. Complete map books, for example, often include pages in addition to the standard reference map pages generated by Data Driven Pages. The use of arcpy.mapping is a necessary step for building complete map books.
This part of the tutorial will simulate the creation of a multipage report. First you will create a new PDF document and then append the PDFs that you created in earlier steps. Imagine that those PDF documents are simply the individual documents that make up a map book. Perhaps Doc1.pdf could be a multipage PDF representing a title page, table of contents, and so on. The second PDF document could be the results of exporting all your Data Driven Pages map pages to a single multipage PDF.
The first step is to create a new PDF document.
- In the Python window, type the following and press ENTER when done:
- In the Python window, type the following three lines, pressing ENTER after each one:
>>> PDFdoc = arcpy.mapping.PDFDocumentCreate(r"C:\Project\Final.pdf")
>>> PDFdoc.appendPages(r"C:\Project\Doc1.pdf")
>>> PDFdoc.appendPages(r"C:\Project\Doc2.pdf")
>>> PDFdoc.saveAndClose()
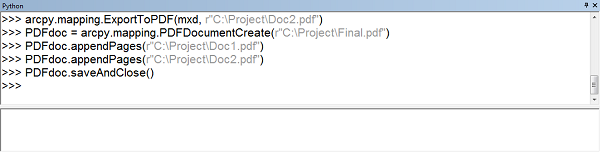
Related topics
You have completed a very simple, yet common workflow using arcpy.mapping. The next steps are to apply these workflows against your own map documents and layers. Also take some time and dig deeper into the arcpy.mapping help topics. There are 100's of small code samples at the ends of each topic that can be easily copied and pasted into the Python window. Also perform a search within the Resource Center on arcpy.mapping to find more advanced arcpy.mapping applications.